The plant watering robot that helps you to never kill your herbs again.
For years on end I tried to grow herbs indoors, only to kill them every single time.
I love plants, but they tend not to love me back.
So, what do I do?
Well, I am a German engineer. So, in accordance with this stereotype, I will build an automated system, that will take care of this for me.
I had this idea for years and I believe now is the time to get a step closer to this little dream of mine.
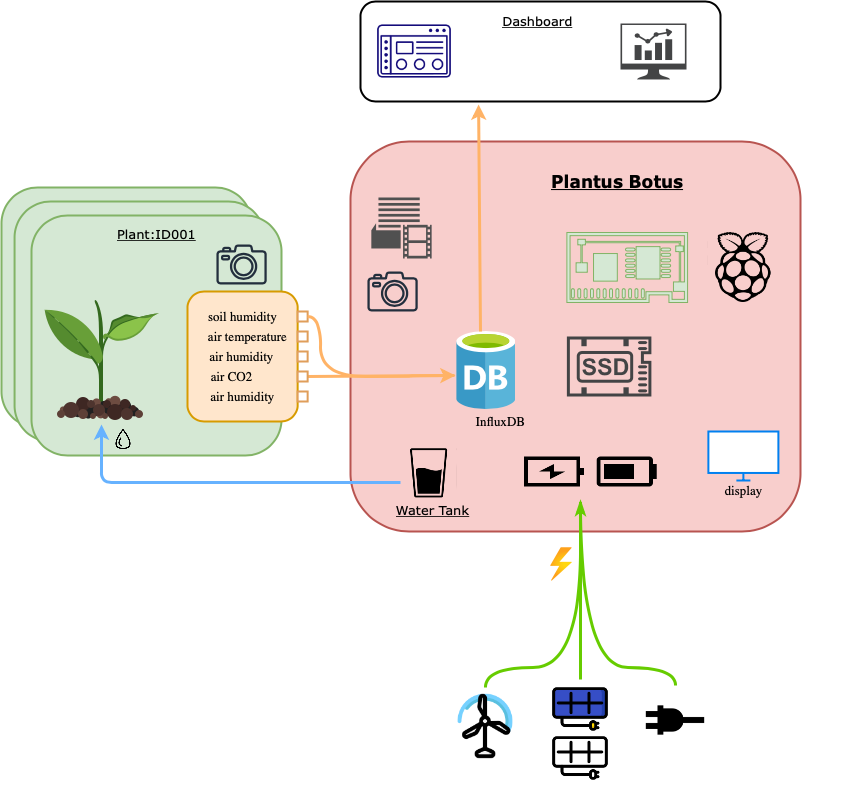
Requirements:
- grow light
- watering system
- humidity sensor in the soil
- database: what herb/plant needs how much water & light
- no theoretical limit, in how many plants can be managed at any time
- Herbs:
- Rosmarin
- Schnittlauch
- Dill
- Petersilie
- Basilikum
- Oregano
- Others
- Chili
Component List
- Raspberry Pi Zero W
- Water
- pumps
- tubes
- water container
- Light
- grow light
- switch
- Sensors
- DockerPi SensorHub [52pi wiki]
- light sensor
- humidity air
- humidity soil
- temperature
- CO2
- ideas
- Sense HAT [raspberrpi.org] – Python Library Doc – berrybase
- Spectrometer GitHub
- remote sensor communication – ESP32, LoRa, MicroPython GitHub
- Camera
- visual -> timelapse
- UV -> analysis & fun
- Power
- Battery
- Battery charging
- Display
- E-Ink display or OLED
- show current parameters
- show graph of parameters
Comments so far:
I thought about not using a Pi, but instead using a ESP32 Microcontroller with MicroPython/CircuitPython, but I just love the RPi and I have unused lying around, so I might just as well use them. Also, maybe I can use it to perform analysis of some plants parameters over time … we will see.
Instruction & Inspirations
- Make a Raspberry Pi Automated Gardener [YouTube@HackerShack]
Amazing project! Pumping water and switching light on/off with RPi Zero W
hackstet.io – GitHub - Raspberry Pi Plant Watering (& Time Lapse) [YouTube@ExplainingComputers]
Pumping water in a bowl of multiple pots, when one pot is dry
blog post – Moisture_Test.py – Valve_Test.py – Watering.py
V0 – SetUp
Log2Ram – Expanding SDLifetime [PiUpLife]
PinOut (interactive)
2022-01-29: currently there seem to be two major libraries for the interaction with the GPIO pins on the Pis:
- gpiozero [readthedocs] (pure Python and based on RPi.GPIO and
- RPi.GPIO [PyPi] –> I used this one
Install GPIO Libraries GPIO python libraries
$ sudo pip3 install gpiozero
[GPIOzero Documentation]$ sudo pip3 install --upgrade RPi.GPIO
I2C
- SMbus2 python library [GitHub@smbus2]
$ pip install smbus2
- Using multiple i2c devices [instructables]
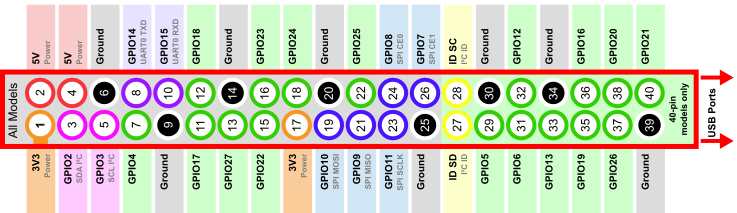
V1 – Starting with the Basics
In the first version, the system should just pump water. It’s sort of a prototype, but if my ambition ends here, it still will be a success for me. I hope for the plants as well …
Start program at system startup [YouTube@Hackster]
$ sudo nvim /etc/rc.local
add
python <full path to script>
- DHT11 temp & air humidity Adafruit Library [GitHub]
- humidity&temperatur DHT-11 [RPi Tutorials]
$ sudo pip3 install adafruit-circuitpython-dht
- old library works: adafruit [GitHub]
$ sudo pip3 install Adafruit_DHT
IG – InfluxDb & Grafana
Install InfluxDB – v1.6.4 – CactusProject – [InfluxData]
Docu Use CLI [influxDocu]
[influxdata community]:
$ sudo apt install influxdb
$ sudo apt install influxdb-client
$ curl "http://localhost:8086/query" --data-urlencode "q=CREATE USER myuser WITH PASSWORD 'mypass' WITH ALL PRIVILEGES"
[stackoverflow]
Trouble Shooting
$ journalctl -e -u influxd -u influxdb
Set logging level: influxDocu
$ nvim /etc/influxdb/influxdb.conf
change logging level from “info” to “debug”
Grafana
- Check for current version: [Grafana]
$ sudo apt install -y adduser libfontconfig1
$ wget https://dl.grafana.com/enterprise/release/grafana-enterprise-rpi_8.3.4_armhf.deb
- $ wget https://
$ sudo dpkg -i grafana-enterprise-rpi_8.3.4_armhf.deb
$ sudo apt update
$ sudo apt install grafana-enterprise-rpi
$ sudo service grafana-server start
$ sudo update-rc.d grafana-server defaults
TroubleShooting
$ sudo systemctl restart grafana-server
$ sudo grafana-cli admin reset-admin-password <new password>
- check that what grafana thinks is NOW is actually NOW
(select past1h and see if the selected time range matches actual UTC reality. In my case it was 2h behind, which was why I could write data to the DB but never actually see any datapoints, since the UTC timestamps were in the future relative to grafana’s reality)
http://raspberrypi.local:3000/login
User: admin – PW: admin
[Grafana] [circuits.dk]
V2 – Using the Database
$ pip install influxdb
2022-06-21 v5.2.0 – but with an unknown input error
from influxdb import InfluxDBClient
#Setup database
client = InfluxDBClient(host='localhost', port=8086, username="admin", password="admin")
client.get_list_database()
# client.create_database('test')
client.switch_database('test')
client.write_points(list_of_dict)
[GitHub] – [YouTube@DevOpsJourney] – [influxDB@gettingStarted] – [influxDB@APIdocs]
V3 – Writing data to the Database
- connect to database
client = InfluxDBClient(host='localhost', port=8086, username="admin", password="admin")
client.switch_database("test")
- collect sensor information as datapoints in a list of dictionaries
sensor_data = [26.0, 48.1]
datapoints = []
data = {
"measurement": "ambient sensor",
"time": datetime.now(),
"fields": {
"temperature": sensor_data[0],
"humidity": sensor_data[1]
}
}
datapoints.append(data)